Math
All node variations

All Math operations have the ability to blend (Mix) between value 1 and the result of the operation
Math
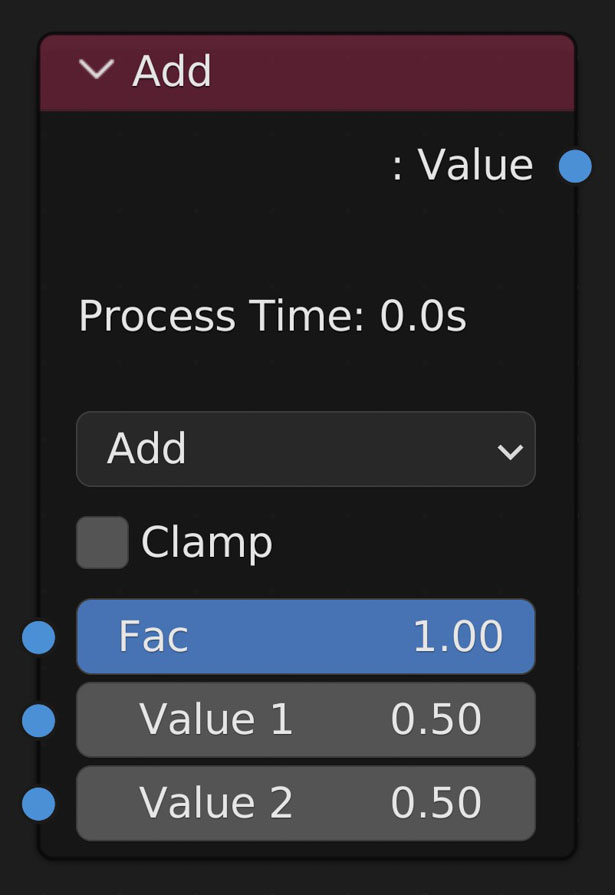
Italicized words are the names of the inputs
Mix
- Blend the value inputs via the Fac(tor) input
Add
- The sum of the two values.
- Value 1 + Value 2
- Ex: 1 + 2 = 3
Subtract
- The difference between the two values
- Value 1 - Value 2
- Ex: 1 - 2 = -1
Multiply
- The product of the two values
- Value 1 * Value 2
- Ex: 3 * 2 = 6
Divide
- The division of the first value by the second value.
- Value 1 / Value 2
- Ex: 6 / 2 = 3
Multiply Add
- The sum of the product of the two values with Addend.
- Value * Multiplier + Addend
Power
- The Base raised to the power of Exponent.
- BaseExponent
- Ex: 32 = 9
Logarithm
- The log of the value with a Base as its base.
- logBase(Value)
- Ex: log(3) = 1.0986122886681098
Square Root
- The square root of the value.
- √Value
- Ex: √4 = 2
Inverse Square Root
- One divided by the square root of the value.
- 1 / √Value
- Ex: 1 / √4 = 0.5
Absolute
- The input value is read with without regard to its sign. This turns negative values into positive values.
- | Value |
- Ex: | -2 | = 2
- Ex: | 5 | = 5
Exponent
- Raises Euler’s number to the power of the value.
- eValue
- e5 = 148.41315910257657
Minimum
- Outputs the smallest of the input values.
- Returns the smallest value min(Value 1, Value 2)
- min(3,7) = 3
- min(10,-8) = -8
Maximum
- Outputs the largest of two input values.
- Returns the largest value max(Value 1, Value 2)
- max(3,7) = 7
- max(10,-8) = 10
Less Than
- Outputs 1.0 if the first value is smaller than the second value. Otherwise the output is 0.0.
- Returns 1 if True, 0 if False Value < Threshold
- 3 < 7 = 1 (True)
- 10 < -8 = 0 (False)
Greater Than
- Outputs 1.0 if the first value is larger than the second value. Otherwise the output is 0.0.
- Returns 1 if True, 0 if False Value > Threshold
- 3 > 7 = 0 (False)
- 10 > -8 = 1 (True)
Sign
- Extracts the sign of Value. All positive numbers will output 1.0. All negative numbers will output -1.0. And 0.0 will output 0.0.
- sign(3) = 1.0
- sign(-50.758) = -1.0
- sign(0) = 0.0
Compare
- Outputs 1.0 if the difference between Value 1 and Value 2 is less than or equal to Epsilon.
- Value 1 = 0.5
- Value 2 = 0.7
- Epsilon = 0.0
- Returns 0.0
- Epsilon = 0.2
- Returns 1.0
Smooth Minimum
- Like the Minimum function but rounded instead of pointed (linear)
- Smooth Minimum
Smooth Maximum
- Like the Maximum function but rounded instead of pointed (linear)
- Smooth Maximum
Round
- Round Value to nearest whole number
- 0.5 = 0.0
- 0.51 = 1.0
- 5.25 = 5.0
- 5.75 = 6.0
Floor
- Round Value down to a whole number
- 0.5 = 0.0
- 0.51 = 0.0
- 5.25 = 5.0
- 5.75 = 5.0
Ceil
- Round Value up to a whole number
- 0.5 = 1.0
- 0.51 = 1.0
- 5.25 = 1.0
- 5.75 = 1.0
Fraction
- Returns everything after the decimal point of Value
- 0.5 = 0.5
- 0.51 = 0.51
- 5.25 = 0.25
- 5.75 = 0.75
Truncate
- Outputs the integer part of the Value
- 0.5 = 0.5
- 0.51 = 0.51
- 5.25 = 0.25
- 5.75 = 0.75
Modulo
- Outputs the remainder once Value 1 is divided by Value 2.
- 5 % 3 = 2
- 5 % 2 = 1
Wrap
- Outputs a value between Min and Max based on the absolute difference between Value and the nearest integer multiple of Max less than the Value.
- Assume Min is 0.0 and Max is 0.1. The result of wrap increase as Value increase. BUT once Value = Max the result goes back to 0.0 and starts over.
- Min = 0.0
- Max = 0.2
- wrap(0.05) = 0.05
- wrap(0.175) = 0.175
- wrap(0.2) = 0.0
- wrap(0.2 + 0.05) = 0.05
- wrap(0.2 + 3.175) = 0.175
Snap
- Round Value down to the nearest integer multiple of Increment.
- floor( Value 1 / Increment ) * Increment
Ping-Pong
- The output value is moved between 0.0 and the Scale based on the input value.
- Like Wrap but with a linear progression back down to 0 instead of instantly at 0
- Scale = 0.2
- ping-pong(0.05) = 0.05
- ping-pong(0.175) = 0.175
- ping-pong(0.2) = 0.2
- ping-pong(0.2 + 0.05) = 0.195
- ping-pong(0.2 + 0.175) = 0.025
Sine
- The Sine of Value
- sin(Value)
Cosine
- The Cosine of Value
- cos(Value)
Tangent
- The Tangent of Value
- tan(Value)
Arcsine
- The Arcsine of Value
- asinine(Value)
Arccosine
- The Arccosine of Value
- acos(Value)
Arctangent
- The Arctangent of Value
- atan(Value)
Arctan2
- Outputs the Inverse Tangent of the first value divided by the second value measured in radians.
- atan2(Value)
Hyperbolic Sine
- The Hyperbolic Sine of Value
Hyperbolic Cosine
- The Hyperbolic Cosine of Value
Hyperbolic Tangent
- The Hyperbolic Tangent of Value
To Radians
- Converts Degrees to radians
To Degrees
- Converts Radians to degrees
Clamp
- Limits the output to be between the Min and Max
- Value: -1, Min: 0, Max: 1 = 0
- Value: 2 Min: 0 Max: 1 = 1
- Value: 0.56 Min: 0 Max: 1 = 0.56
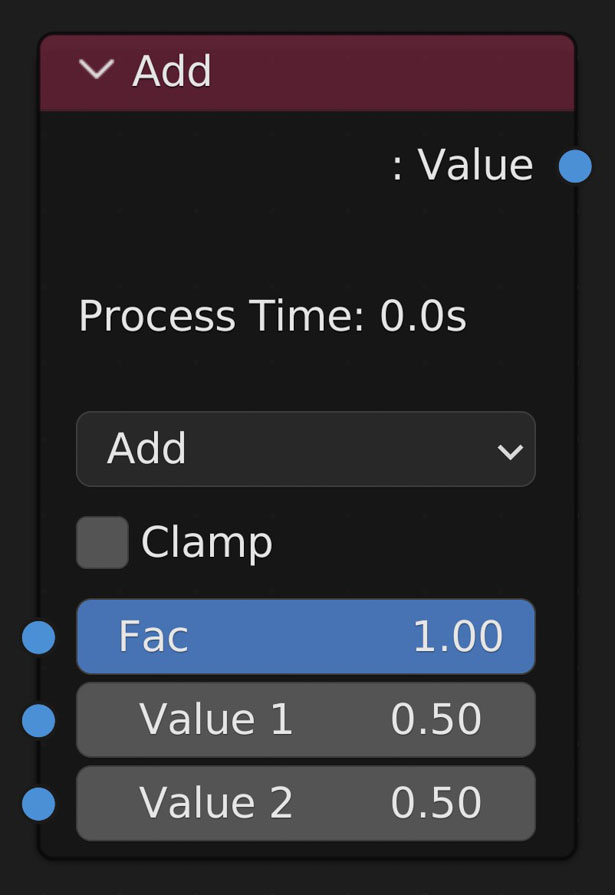
Italicized words are the names of the inputs
Mix
- Blend the value inputs via the Fac(tor) input
Add
- The sum of the two values.
- Value 1 + Value 2
- Ex: 1 + 2 = 3
Subtract
- The difference between the two values
- Value 1 - Value 2
- Ex: 1 - 2 = -1
Multiply
- The product of the two values
- Value 1 * Value 2
- Ex: 3 * 2 = 6
Divide
- The division of the first value by the second value.
- Value 1 / Value 2
- Ex: 6 / 2 = 3
Multiply Add
- The sum of the product of the two values with Addend.
- Value * Multiplier + Addend
Power
- The Base raised to the power of Exponent.
- BaseExponent
- Ex: 32 = 9
Logarithm
- The log of the value with a Base as its base.
- logBase(Value)
- Ex: log(3) = 1.0986122886681098
Square Root
- The square root of the value.
- √Value
- Ex: √4 = 2
Inverse Square Root
- One divided by the square root of the value.
- 1 / √Value
- Ex: 1 / √4 = 0.5
Absolute
- The input value is read with without regard to its sign. This turns negative values into positive values.
- | Value |
- Ex: | -2 | = 2
- Ex: | 5 | = 5
Exponent
- Raises Euler’s number to the power of the value.
- eValue
- e5 = 148.41315910257657
Minimum
- Outputs the smallest of the input values.
- Returns the smallest value min(Value 1, Value 2)
- min(3,7) = 3
- min(10,-8) = -8
Maximum
- Outputs the largest of two input values.
- Returns the largest value max(Value 1, Value 2)
- max(3,7) = 7
- max(10,-8) = 10
Less Than
- Outputs 1.0 if the first value is smaller than the second value. Otherwise the output is 0.0.
- Returns 1 if True, 0 if False Value < Threshold
- 3 < 7 = 1 (True)
- 10 < -8 = 0 (False)
Greater Than
- Outputs 1.0 if the first value is larger than the second value. Otherwise the output is 0.0.
- Returns 1 if True, 0 if False Value > Threshold
- 3 > 7 = 0 (False)
- 10 > -8 = 1 (True)
Sign
- Extracts the sign of Value. All positive numbers will output 1.0. All negative numbers will output -1.0. And 0.0 will output 0.0.
- sign(3) = 1.0
- sign(-50.758) = -1.0
- sign(0) = 0.0
Compare
- Outputs 1.0 if the difference between Value 1 and Value 2 is less than or equal to Epsilon.
- Value 1 = 0.5
- Value 2 = 0.7
- Epsilon = 0.0
- Returns 0.0
- Epsilon = 0.2
- Returns 1.0
Smooth Minimum
- Like the Minimum function but rounded instead of pointed (linear)
- Smooth Minimum
Smooth Maximum
- Like the Maximum function but rounded instead of pointed (linear)
- Smooth Maximum
Round
- Round Value to nearest whole number
- 0.5 = 0.0
- 0.51 = 1.0
- 5.25 = 5.0
- 5.75 = 6.0
Floor
- Round Value down to a whole number
- 0.5 = 0.0
- 0.51 = 0.0
- 5.25 = 5.0
- 5.75 = 5.0
Ceil
- Round Value up to a whole number
- 0.5 = 1.0
- 0.51 = 1.0
- 5.25 = 1.0
- 5.75 = 1.0
Fraction
- Returns everything after the decimal point of Value
- 0.5 = 0.5
- 0.51 = 0.51
- 5.25 = 0.25
- 5.75 = 0.75
Truncate
- Outputs the integer part of the Value
- 0.5 = 0.5
- 0.51 = 0.51
- 5.25 = 0.25
- 5.75 = 0.75
Modulo
- Outputs the remainder once Value 1 is divided by Value 2.
- 5 % 3 = 2
- 5 % 2 = 1
Wrap
- Outputs a value between Min and Max based on the absolute difference between Value and the nearest integer multiple of Max less than the Value.
- Assume Min is 0.0 and Max is 0.1. The result of wrap increase as Value increase. BUT once Value = Max the result goes back to 0.0 and starts over.
- Min = 0.0
- Max = 0.2
- wrap(0.05) = 0.05
- wrap(0.175) = 0.175
- wrap(0.2) = 0.0
- wrap(0.2 + 0.05) = 0.05
- wrap(0.2 + 3.175) = 0.175
Snap
- Round Value down to the nearest integer multiple of Increment.
- floor( Value 1 / Increment ) * Increment
Ping-Pong
- The output value is moved between 0.0 and the Scale based on the input value.
- Like Wrap but with a linear progression back down to 0 instead of instantly at 0
- Scale = 0.2
- ping-pong(0.05) = 0.05
- ping-pong(0.175) = 0.175
- ping-pong(0.2) = 0.2
- ping-pong(0.2 + 0.05) = 0.195
- ping-pong(0.2 + 0.175) = 0.025
Sine
- The Sine of Value
- sin(Value)
Cosine
- The Cosine of Value
- cos(Value)
Tangent
- The Tangent of Value
- tan(Value)
Arcsine
- The Arcsine of Value
- asinine(Value)
Arccosine
- The Arccosine of Value
- acos(Value)
Arctangent
- The Arctangent of Value
- atan(Value)
Arctan2
- Outputs the Inverse Tangent of the first value divided by the second value measured in radians.
- atan2(Value)
Hyperbolic Sine
- The Hyperbolic Sine of Value
Hyperbolic Cosine
- The Hyperbolic Cosine of Value
Hyperbolic Tangent
- The Hyperbolic Tangent of Value
To Radians
- Converts Degrees to radians
To Degrees
- Converts Radians to degrees
Clamp
- Limits the output to be between the Min and Max
- Value: -1, Min: 0, Max: 1 = 0
- Value: 2 Min: 0 Max: 1 = 1
- Value: 0.56 Min: 0 Max: 1 = 0.56
No items found.
Created:
Sunday, August 6, 2023
updated:
Wednesday, August 9, 2023
True-VFX
Author |
Heather @ True-VFX